operations
Every child of crud_objects must contain an operations object. In turn, this object contains custom objects that you define, each of which specifies a CRUD call related to the relevant system object type.
The name of each custom object inside operations should be a simple word or words separated by an underscore (_) or dash (-) that names the CRUD call. For example: users_get
, user_create
, user_delete
, user_modify_mail
, etc. Each custom object can take a variety of properties, which are listed below.
Operation names show up in the NIM Studio as names of Functions in Mappings:
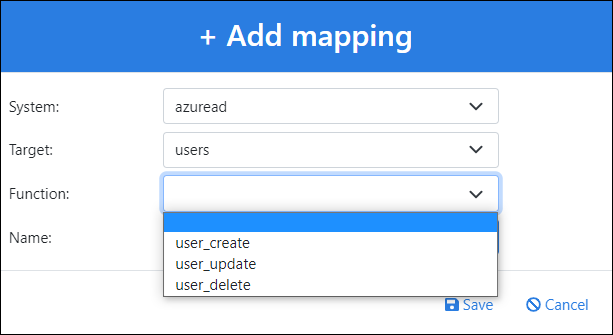
For most external systems, you will want to specify the full range of CRUD operations for each object (get, create, update, delete), but this is not strictly required.
get
get operations are specified somewhat differently than post/put/patch/delete operations, and support the following properties. A REST system's get calls are used during Collection.
Tip
You should always specify get operations for all relevant system object types, regardless of whether the system is a target or only a source (see Sources and targets).
method
The REST method. For get calls, must be get
. There should be only a single get operation for each type of object in the external system (i.e., for each child object of crud_objects).
call
Optional. The call object of get specifies details of the HTTP call.
Two call modes are supported:
mode=normal
Use the normal mode to make a single sequence of paged calls to the external system. This is the most common scenario.
"call": { "mode": "normal", "path": "/groups/{id}/aliases" },
The path property is optional. If specified, it is appended to the baseUrl to build the full URL for the call. If it is not specified, the name of the parent object under crud_objects) is used instead. The path property can contain variables.
mode=iteration
Use the iteration mode when the relevant data table must be populated with a separate REST call per row of a different table (table).
The most common use case is group membership collection. In NIM, all members of all groups are stored in a single table, but most REST systems only support collection of members for one group at a time. Thus, one call must be made per group. The iteration mode is the solution.
"members": { "operations": { "members_get": { "method": "get", "call": { "mode": "iteration", "table": "groups", "iterator": "id", "path": "/groups/{iterator}/members", "base": { "group_id": "{iterator}" } }, "maxSessionCount": 20 }
When using the iteration mode, configure the properties of call as follows:
- mode
Set to
iteration
.- table
The table for which a call will be made per row. Set this to the name of another child object of crud_objects. For this example (the
members
table), it is thegroups
table.- iterator
The resource (column) of the table which will be used to uniquely identify the calls and results. Typically, this is the name of the table's key resource. For this example, it is the
id
resource of thegroups
table.- path
The path for the get request. Must include the {iterator} variable somewhere in the path, per the specific requirements of the external system.
- base
Each child property specified in base becomes a column in the result table (the table into which the iterated call results are written). For this example, we're adding a
group_id
column whose value is equal to {iterator}. This is necessary to identify the group in the results. The base object should always contain the {iterator} variable. The property must be defined under resources to populate in NIM
maxPageCount
Optional. Overrides the default maxPageCount setting in the rest object. Only used by Azure AD and Google systems. All generic systems (see call_handling) instead use pagination.
maxSessionCount
The maximum number of concurrent get requests when using mode=iteration. When the maximum is reached, NIM will wait for all calls to return before the next set of calls is executed.
processing_options
Optional. This object specifies how to interpret the result data.
The output_field property specifies where to find the result data. In the example shown, the result objects can be found in the section child property of the sections object.
The stringArrayColumnName is used if the response is an array of strings instead of an array of objects. The value should be the name of a string
type specifier in the resources object of the current crud_object. The result data will be stored in that column.
"processing_options": { "output_field": "sections.section" "stringArrayColumnName": "id" }
If the result data is directly inside the result object, a value of null
must be specified:
"processing_options": { "output_field": "null" "stringArrayColumnName": "id" }
query_parameters
Optional. Specifies additional query_parameters for the get request.
verb
Optional. Only used in cases where the target system expects an HTTP verb other than get for get calls. For example, some external systems expect post instead.
post/put/patch/delete
The post/put/patch/delete operations are used to write into external systems used in target contexts. You should not specify any post/put/patch/delete operations for external systems that will only be used in source contexts (see Sources and targets).
These operations are very similar, with a few exceptions. Each operation is specified by a single JSON object that contains the following properties:
method
The method is determined by the requirements of the REST target system and the call you're specifying. It is either post, put, patch, or delete.
body
Calls of type post, put and patch can optionally have a body. If no body property is specified explicitly, it will be composed of the specified resources. If a body property is specified, it acts as a template. It can contain variables that refer to resources.
Example JSON:
"member_add": { "method": "post", "body":{ "@odata.id":https://graph.microsoft.com/v1.0/directoryObjects/{id}" }
call
The optional call specification is similar to the mode=normal configuration of get calls. Only the mode=normal configuration is supported for post/put/patch/delete calls.
Example JSON:
"user_create": { "method": "post", "call": { "mode": "normal", "path": "/users", }
semantics
The semantics with which to process the call results. Possible values include:
create
delete
update
set
memberships-update
resource_allowance_default
Specifies the allowance setting for resources that are not explicitly listed in resource_mandatory or resource_prohibited. Possible values include:
optional
prohibited
mandatory
Example JSON:
"resource_allowance_default": "optional",
resource_prohibited
A list of resources that cannot be specified for this operation.
Example JSON:
"resource_prohibited": [ "id", "createdDateTime", "renewedDateTime" ]
resource_mandatory
A list of resources that must be specified for this operation.
Example JSON:
"resource_mandatory": [ "displayName", "mailEnabled", "mailNickname", "securityEnabled" ]
warning_only
Error codes that should only generate a warning.
For example, if a 409 is returned when removing a non-existent member from a group:
"warning_only": [ 409 ]